Monitoring Earth for asteroid collisions with Dataristix®
While not a task usually performed in industrial settings, this example of monitoring potentially hazardous near-Earth objects touches on various aspects of Dataristix and may serve as a guideline for more mundane applications in your corner of the globe.
Near-Earth object data is is made available by NASA via a public REST API. The task for Dataristix is therefore, to call the REST API in regular intervals, extract the response, and evaluate indicators that mark objects as "hazardous". We then send an e-mail to warn recipients about a potential collision with Earth, identifying the dangerous asteroids.
What we'll need for the example is the Dataristix Connector for REST to communicate with NASA, the Dataristix Connector for Script for some response parsing, and the Dataristix Connector for E-Mail to send some notifications. If you have these connector modules already installed, then please check Dataristix for updates to ensure that you have the latest version.
Warning: We cannot take responsibility should you collide with an asteroid without prior notice. Follow this example at your own risk.
NASA REST API
NASA's API may be called with a demo API key for less frequent requests. Since our monitoring solution does not need to refresh quickly, we'll just be using the demo key. We'll be requesting data once a day only and pass the date as the start and end date to the API. For the 9th of November 2024, the request URL looks like this:
https://api.nasa.gov/neo/rest/v1/feed?start_date=2024-11-09&end_date=2024-11-09&api_key=DEMO_KEY
The response up to the first object returned is as follows.
{ "links": { "next": "http://api.nasa.gov/neo/rest/v1/feed?start_date=2024-11-10&end_date=2024-11-10&detailed=false&api_key=DEMO_KEY", "prev": "http://api.nasa.gov/neo/rest/v1/feed?start_date=2024-11-08&end_date=2024-11-08&detailed=false&api_key=DEMO_KEY", "self": "http://api.nasa.gov/neo/rest/v1/feed?start_date=2024-11-09&end_date=2024-11-09&detailed=false&api_key=DEMO_KEY" }, "element_count": 19, "near_earth_objects": { "2024-11-09": [ { "links": { "self": "http://api.nasa.gov/neo/rest/v1/neo/3408650?api_key=DEMO_KEY" }, "id": "3408650", "neo_reference_id": "3408650", "name": "(2008 GL20)", "nasa_jpl_url": "https://ssd.jpl.nasa.gov/tools/sbdb_lookup.html#/?sstr=3408650", "absolute_magnitude_h": 26.6, "estimated_diameter": { "kilometers": { "estimated_diameter_min": 0.0127219879, "estimated_diameter_max": 0.0284472297 }, "meters": { "estimated_diameter_min": 12.7219878539, "estimated_diameter_max": 28.4472296503 }, "miles": { "estimated_diameter_min": 0.0079050743, "estimated_diameter_max": 0.0176762835 }, "feet": { "estimated_diameter_min": 41.7388066307, "estimated_diameter_max": 93.330808926 } }, "is_potentially_hazardous_asteroid": false, "close_approach_data": [ { "close_approach_date": "2024-11-09", "close_approach_date_full": "2024-Nov-09 01:42", "epoch_date_close_approach": 1731116520000, "relative_velocity": { "kilometers_per_second": "9.1729569273", "kilometers_per_hour": "33022.6449382016", "miles_per_hour": "20518.9873506287" }, "miss_distance": { "astronomical": "0.2789515808", "lunar": "108.5121649312", "kilometers": "41730562.320812896", "miles": "25930169.0282381248" }, "orbiting_body": "Earth" } ], "is_sentry_object": false },
...
Important to note is, that the start date (which is also the end date in our case) is encoded as a property name of "near_earth_objects" rather than a property value. This means, we cannot use JSON paths to extract the near-Earth objects array that follows. This can be dealt with easily though by adding some additional task logic utilizing the free Script Connector. As we will see, the Script Connector can be a convenient option when task node logic becomes complex.
Configure a REST API client
We start with adding a REST client, name it "NASA NEO" and configure it as follows.
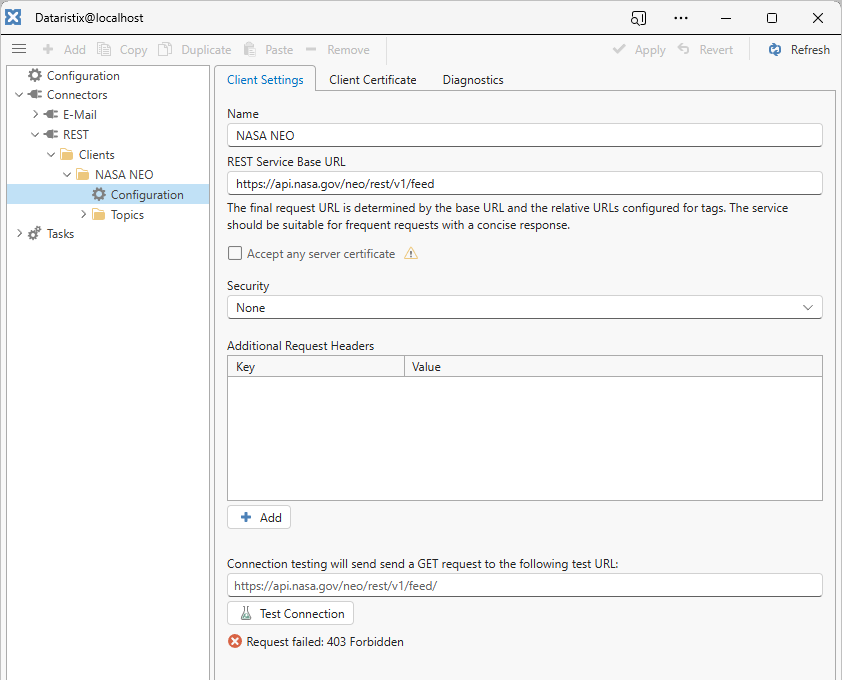
We set the service base URL to https://api.nasa.gov/neo/rest/v1/feed
. The base URL itself cannot contain query parameters. We leave the Security option as "None", and show how to pass the API key, start date, and end date as query parameters below. Testing the connection for the base URL results in error "Forbidden". This is the expected response in this case, because we have not defined required parameters yet.
After setting up the REST API client this way, click on the toolbar's "Apply" button to save the configuration.
Configure a REST API topic
We'll now add a topic to define how we want to fetch data. We call the topic "NEOs". Topics can either have "Read" access, "Write" access, or "Process" access. Because we'll need to pass some parameters to the query and expect a result, we'll mark the topic access as "Process". This allows us to add request parameter names "start_date", "end_date", and "api_key" for later use in the task logic. Press the "Apply" toolbar button to commit changes.
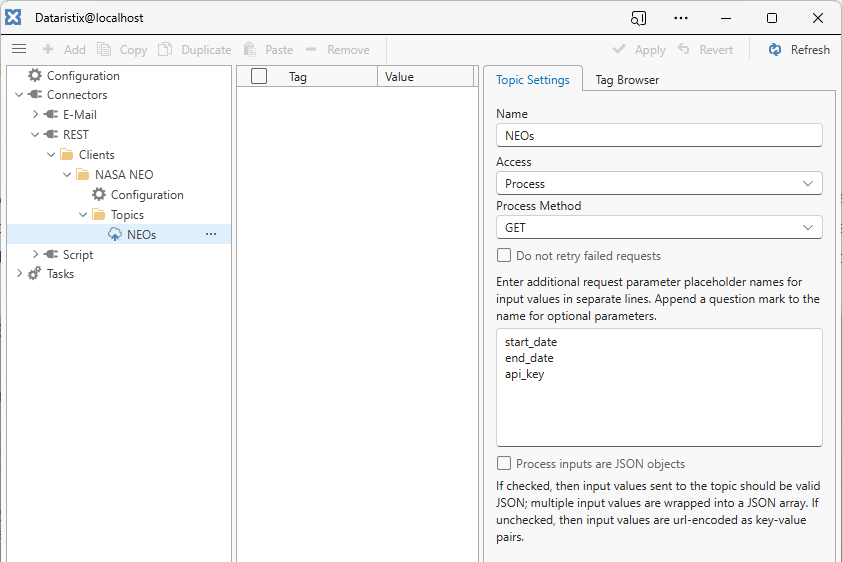
Next, with the "NEOs" topic selected, we'll press the "Add" toolbar button to add an output tag that will give us access to the API response. Leave the tag name prefix empty and simply enter "Result" as the only tag name.
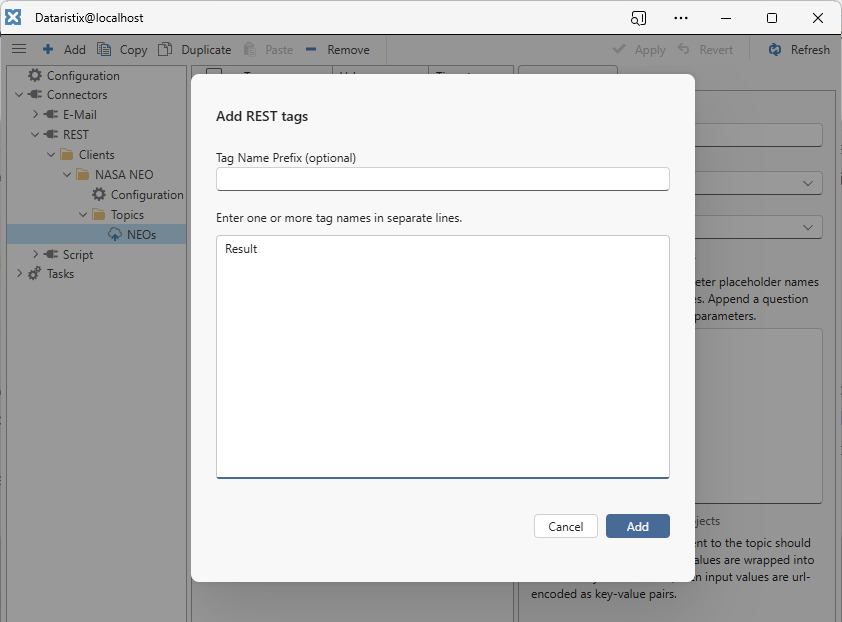
We don't define any relative URL or JSON path for the "Result" tag because we plan to parse the entire response using the Script Connector.
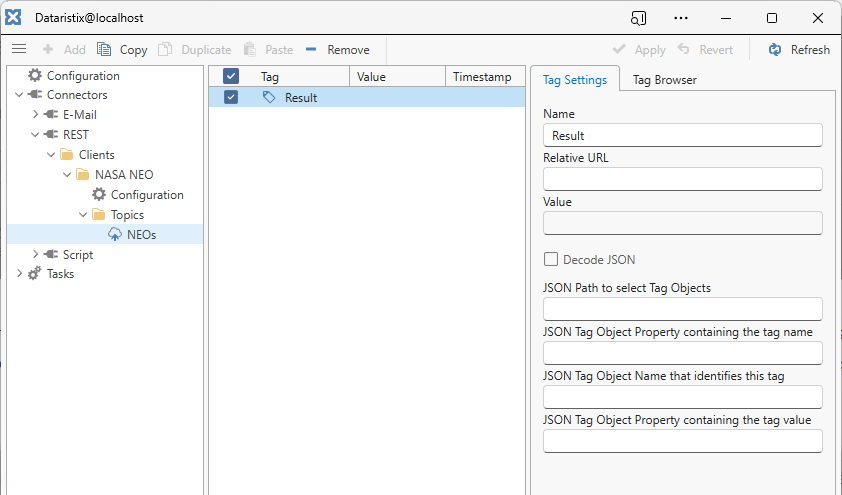
Configure a Script topic
Next up is the Script Connector. We need some JavaScript that parses the NASA API response and constructs the message text that we want to send via e-mail. The script takes a date string in ISO format (i.e., "2024-11-08") as an input parameter, extracts the near-Earth-object array from JSON, and constructs a string listing all hazardous object names and reference URLs as the output.
// Parse near-Earth-objects and return a list of hazardous objects
// in separate lines as a string
const startAndEndDate = inputValues['StartAndEndDate'];
const neoJson = inputValues['NeoJson'];
const o = JSON.parse(neoJson);
// Loop through neos and collect hazardous objects
let neoArray = o.near_earth_objects[startAndEndDate];
let hazardousNeos = [];
neoArray.forEach((elem => {
if (elem.is_potentially_hazardous_asteroid) {
hazardousNeos.push(elem.name + ", " + elem.nasa_jpl_url);
}
}));
// Return string containing hazardous objects
if (hazardousNeos.length > 0) {
outputValues['HazardousNeos'] = hazardousNeos.join('\n');
} else {
outputValues['HazardousNeos'] = "No hazardous objects found.";
}
The Script topic is configured as follows, with specific input tag names "StartAndEndDate" and "NeoJson" (accessible via the predefined "inputValues" variable) and a specific output tag name "HazardousNeos" (accessible via the predefined "outputValues" variable). Input and output tags will be available for tag connections within the task logic.
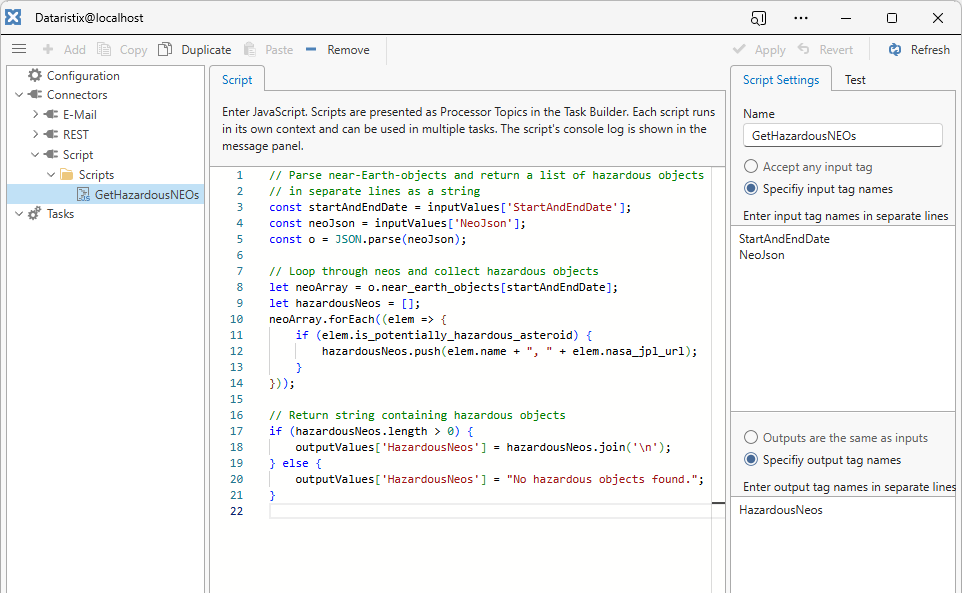
Configure an E-Mail topic
Once we have detected hazardous objects or have determined that there are none, we want to send an e-mail message to tell someone about it. We assume here that the E-Mail connector is already configured for connection with your SMTP provider. What's left to do is adding an E-Mail Connector topic and configuring a message template:
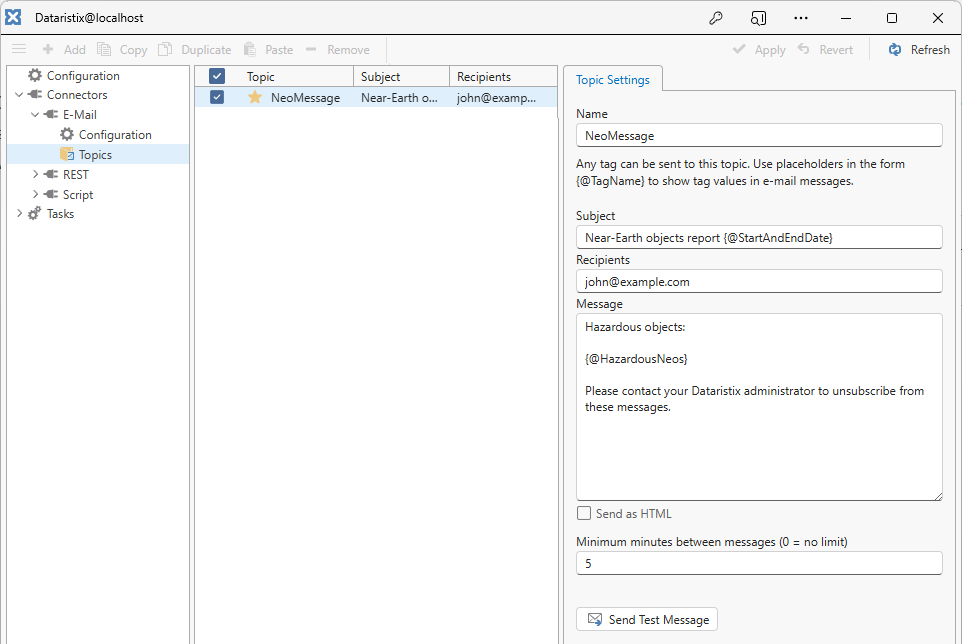
The message template contains placeholders for tags "StartAndEndDate" and "HazardousNeos". If matching tags are connected to the E-Mail topic within the task logic, then placeholders will be replaced with tag values before sending the message.
Configure the monitoring task
We are now ready to configure the task pipeline defining the data flow. Right-click on the "Tasks" node in the navigation panel to add a task, then use the "Build" panel on the right to add required task nodes. Here we call the task "NEO Monitoring".
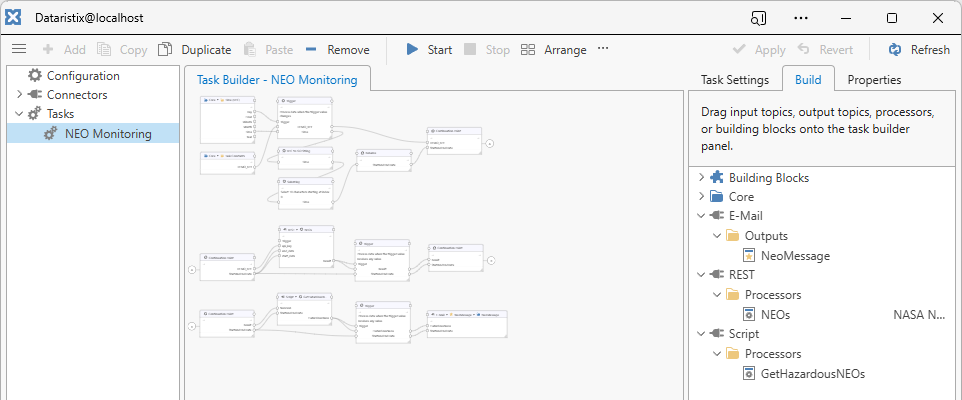
In detail, the task pipeline is made up as follows.
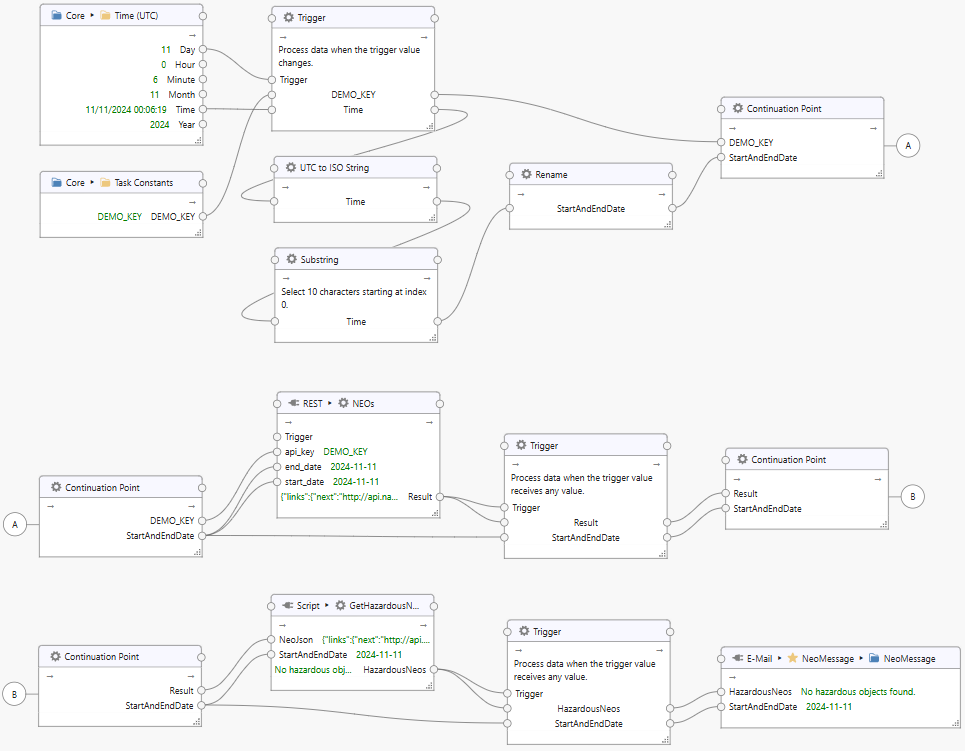
Inputs are the current Time (UTC) and a Task Constant containing the API key. The Trigger connected to the Time and API Key ensures that data is processed once a day. The time input is converted to a date-only ISO string as required by the NASA API, renamed to "StartAndEndDate" for clarity, and, together with the API key, passed on to Continuation Point A. The continuation point in this instance breaks up the task pipeline to be more readable, but it also merges the task pipeline paths so that the API key and "StartAndEndDate" are forwarded at the same time.
Continuing on, API key and "StartAndEndDate" are sent to the REST Connector's "NEOs" topic to call the NEO API. The subsequent Trigger ensures that the pipeline continues processing only after a response is received. The response, together with the "StartAndEndDate", is then passed onto Continuation Point B.
Next, response and "StartAndEndDate" are fed into the "GetHazardousNeos" script to extract a list of potentially hazardous objects as text. Again a trigger is used to ensure that processing continues only after the script result is received.
Finally, the "HazardousNeos" message text and the "StartAndEndDate" are sent to the E-Mail Connector to construct a message, substituting placeholders with actual values.
Start the task using the toolbar button to begin receiving monitoring reports.
On the 12th of November 2024 you may receive a message like this:
Subject: Near-Earth objects report 2024-11-12
Hazardous objects:
413577 (2005 UL5), https://ssd.jpl.nasa.gov/tools/sbdb_lookup.html#/?sstr=2413577
(2006 AM4), https://ssd.jpl.nasa.gov/tools/sbdb_lookup.html#/?sstr=3311964
(2011 VU5), https://ssd.jpl.nasa.gov/tools/sbdb_lookup.html#/?sstr=3586027
Please contact your Dataristix administrator to unsubscribe from these messages.
Further improvements
In the example above, potentially hazardous near-Earth objects are reported on the day when they reach their closest distance to Earth before moving along their orbits for another close encounter in the future. The closest distance on the given day may still be far away from Earth, without any likeliness of a collision. As such, there is probably no need to rush your cup of tea or coffee when an e-mail notification pops up. You may want to consider evaluating the closest distance numbers that are part of the NASA API response and restrict notifications further so that, for example, an e-mail is only sent when an object gets closer than a certain distance. You may also want to run reports for a date a few days in the future for an earlier "heads-up". Some additional error handling could be useful, i.e. when the API call does not return a result. We'll leave these improvements to you as an excercise.
Final thought
(This is the final thought for the article, not generally; we are not aware of any imminent collisions.) While possibly not saving humankind, this example will hopefully provide some inspiration for applying Dataristix to other problem scenarios. If you would like to comment or would like to see some other examples published here, then please contact us, we'd love to receive your feedback.